Python – Upload and Download files Google Cloud Storage

Today in this article we shall see how to use Python – Upload and Download files Google Cloud Storage.
We shall be using the Python Google storage library to upload files and also download files.
Today in this article, we will cover below aspects,
Getting Started
Create any Python application.
Add below Python packages to the application,
Using CLI
pip3 install google-cloud-storage
Additionally if needed,
pip install --upgrade google-cloud-storage
Alternatively,
Using Requirements.txt
google-cloud-storage == 1.28.1
Or you can use the setup.py file to register the dependencies as explained in the below article,
Please add the below namespace to your Python files,
from google.cloud import storage
Below is a sample example for creating New Bucket storage,
Upload a file to Google Cloud Storage using Python
Below is a sample example for uploading a file to Google Cloud Storage.
We shall be uploading sample files from the local machine “CloudBlobTest.pdf” to Google Cloud storage.
def upload_blob(bucket_name, source_file_name, destination_blob_name):
"""Uploads a file to the google storage bucket."""
storage_client = storage.Client()
bucket = storage_client.bucket(bucket_name)
blob = bucket.blob(destination_blob_name)
blob.upload_from_filename(source_file_name)
print(
"File {} uploaded to Storage Bucket with Bob name {} successfully .".format(
source_file_name, destination_blob_name
)
)
The above code will upload the blob with the name “CloudBlobTest.pdf” to the Google Cloud storage location “thecodebuzz“.

Download a file from Google Storage Bucket using Python
Blob can be downloaded from Google Storage Bucket as a file easily by using the Python storage library methods as explained below.
The sample example demonstrates how to download a file from the Google Cloud storage bucket to the local machine file path.
Let’s download the above STEP 1 uploaded file i.e. CloudBlobTest.pdf from the GCS bucket.
We shall try the downloaded blob from the GCS location to below output path which is on the local machine,
@"C:\Test\gcp\cloud\CloudBlobTest_Out.pdf";
def download_blob(bucket_name, source_blob_name, destination_file_name):
"""Downloads a blob."""
storage_client = storage.Client()
bucket = storage_client.bucket(bucket_name)
blob = bucket.blob(source_blob_name)
blob.download_to_filename(destination_file_name)
print(
"Blob {} downloaded to file path {}. successfully ".format(
source_blob_name, destination_file_name
)
)
Once executed the file will be downloaded to the local path.
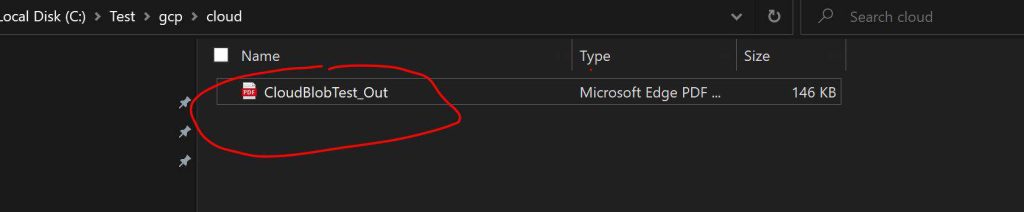
Do you have any comments or ideas or any better suggestions to share?
Please sound off your comments below.
Happy Coding !!
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.