Python – MongoDB Date Time query with examples

Today, in this article will see how to write a MongoDB Date Time query to get records based on timestamp or date range using Python language.
We will use the PyMongo package to install the driver and query MongoDB.
We will cover the below aspects in today’s article,
Getting started
Here below is a sample schema or document we shall use for the date range query,
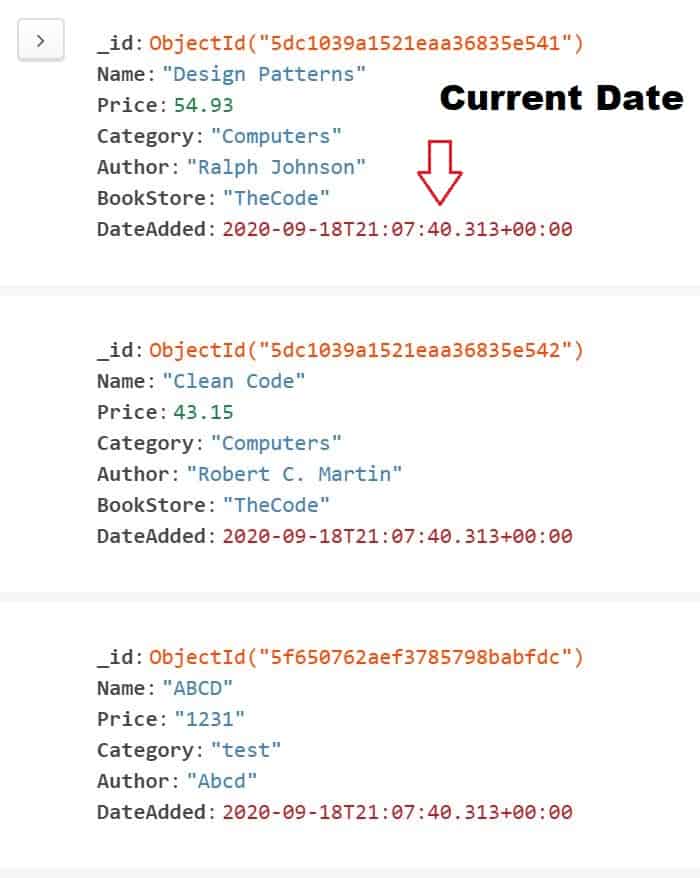
Install PyMongo package
Please install the PyMongo driver package to get support for the MongoClient connection class and properties.
For more details visit- https://api.mongodb.com/python/current
Add PyMongo Import statements
Please add below using namespaces
from pymongo import MongoClient from datetime import datetime
If you need support for date time zone support like UTC or ETC etc, please add below other import statements,
from datetime import datetime, tzinfo, timezone
Define MongoDB Connection
client = MongoClient('mongodb://localhost:27017/')
db = client['your_database']
collection = db['your_collection']
Here we will be using the below query to get the documents between two dates in MongoDB Collection.
Python – Mongo Date greater than query (‘gt’)
Define the date for the query
start_date = datetime(2023, 1, 1)
OR
Date time using time zone
start_date = datetime(2023, 1, 1, tzinfo=timezone.utc)
Define the filter
filter = {
'DateAdded': {
'$gt': start_date
}
}
Get the results
result = client['TheCodeBuzz']['Books'].find(filter=filter)
#print the results
for document in result:
print(document)
Below is the complete code,
from datetime import datetime, tzinfo, timezone
from pymongo import MongoClient
# Define Python Mongo Connection
client = MongoClient('mongodb://localhost:27017/')
db = client['your_database']
collection = db['your_collection']
# define date for the query
start_date = datetime(2023, 1, 1)
# define filter for the query
filter = {
'DateAdded': {
'$gt': start_date
}
}
#execute query
result = client['TheCodeBuzz']['Books'].find(filter=filter)
#print the results
for document in result:
print(document)
Mongo Atlas UI
The above query produces the same result as indicated below using ATLAS UI,
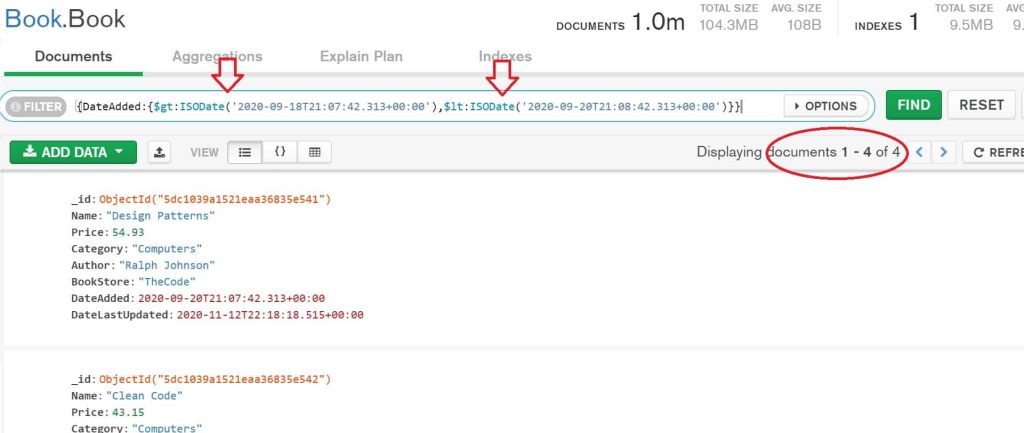
Python – Mongo Date less than query (‘lt’)
# define date for the query
start_date = datetime(2023, 1, 1)
# define filter for the query
filter = {
'DateAdded': {
'$lt': start_date
}
}
Python – Mongo Date less than and greater than date query
# define date for the query
start_date = datetime(2023, 8, 1)
end_date = datetime(2024, 1, 1)
# define filter for the query
filter = {
'DateAdded': {
'$gte': start_date,
'$lt': end_date
}
}
That’s all! Happy coding!
Does this help you fix your issue?
Do you have any better solutions or suggestions? Please sound off your comments below.
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.