File logging in the Middleware in ASP.NET Core
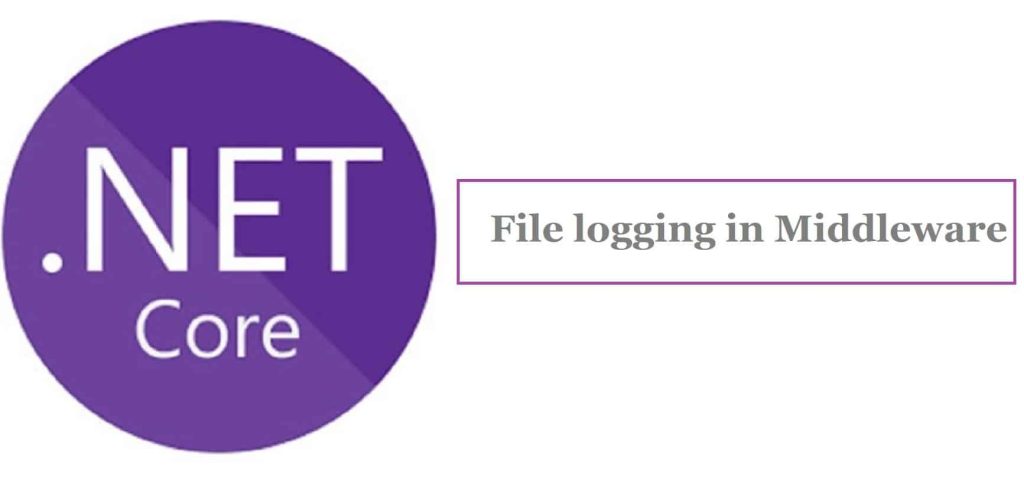
In this article, we will see how to perform File logging in the Middleware in ASP.NET Core application.
As we know Middleware is a component that is used in the application pipeline to handle requests and responses which can also help perform pre and post-operation or address cross-cutting concerns within the API pipeline development.
Today in this article, we will cover below aspects,
We shall use the approach of the middleware component for handling ILoggerFactory within the ASP.NET Core API pipeline.
You can use the above technique of file logging using Serilog or Log4Net or NLog etc.
Getting Started
Let’s create an ASP.NET Core 3.1 application.
Let’s create a very basic middleware component as below,
Create Middleware component
Lets create CustomMiddleware class as below,
public class CustomMiddleware { private readonly RequestDelegate _next; private readonly ILoggerFactory _loggerFactory; public CustomMiddleware(RequestDelegate next, ILoggerFactory loggerFactory) { _next = next; _loggerFactory = loggerFactory; }
Lets now add InvokeAsync(Assuming we need asynchronous middleware behaviour) method.
Create logger instance using CreateLogger method as below,
public async Task InvokeAsync(HttpContext context)
{
var _logger = _loggerFactory.CreateLogger<CustomMiddleware>();
try
{
_logger.LogInformation("Performing file logging in Middleware operation");
// Perform some Database action into Middleware
_logger.LogWarning("Performing Middleware Save operation");
//Save Data
_logger.LogInformation("Save Comepleted");
await _next(context);
}
catch (Exception ex)
{
_logger.LogError($"Something went wrong: {ex.Message}");
}
}
Logging Configuration for LoggerFactory
Once you add File logging using Serilog or NLog or Log4NET your logging start using the configuration you provided.
LoggerFactory shall use the configuration as specified.
For example if using Serilog , you can specify logging configuration using the below techniques,
public static void Main(string[] args)
{
Log.Logger = new LoggerConfiguration()
.WriteTo.File("Thecodebuzz-log-{Date}.txt")
.CreateLogger();
CreateHostBuilder(args).Build().Run();
Log.CloseAndFlush();
}
Please see for more details on File logging using Serilog
The above produces below logging,

That’s All! Happy Coding!
References :
Do you see any improvement to the above code? Do you have any suggestions or thoughts? Please sound off your comments below.
Summary
Today article in this article, we saw how to perform File or Rolling File-based logging in the Middleware component in the ASP.NET Core application. We understood using the Loggerfactory interface we can log to any source including file or rolling file easily.
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.