NSwag API Versioning using Swagger -Guidelines

In this post, we’ll look at how to add NSwag API Versioning using Swagger, also known as OpenAPI versioning, to the API documentation in ASP.NET Core.
NSwag is a standalone library that allows you to generate Swagger specifications and API client code from existing ASP.NET Core controllers or TypeScript code. It provides a robust set of features for both API documentation generation and API client generation. Here are some key aspects of NSwag.
NSwag API Versioning can be enabled using NSwag and related packages for .NET Core APIs, supporting either Swagger V2.0 or OpenAPI V3.0 specifications.
In today’s article, we shall cover below,
Getting started
Step1 :
Let’s create ASP.NET Core API using ASP.NET Core 3.1 or .NET 6,
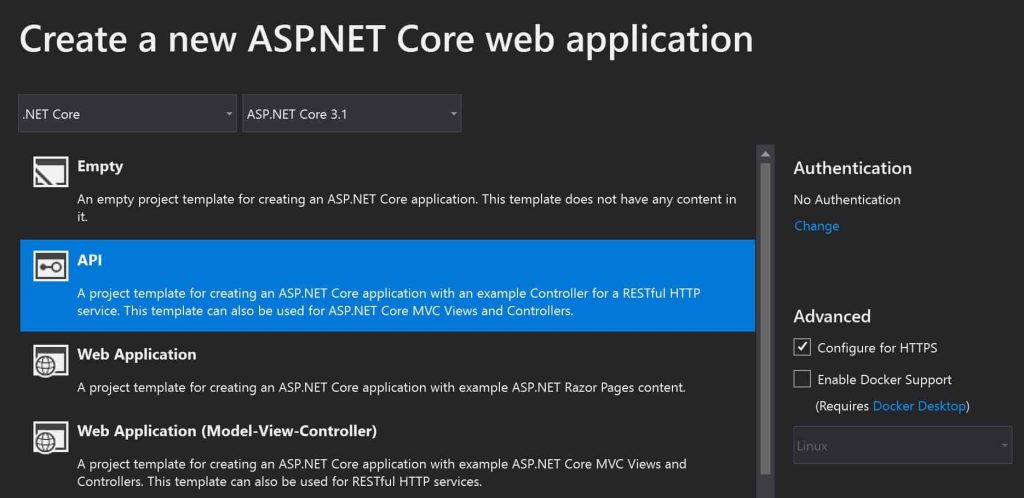
Please install below NuGet package,
PM> Install-Package Microsoft.AspNetCore.Mvc.Versioning -Version <version>
Or
Install from NuGet Package Manager,
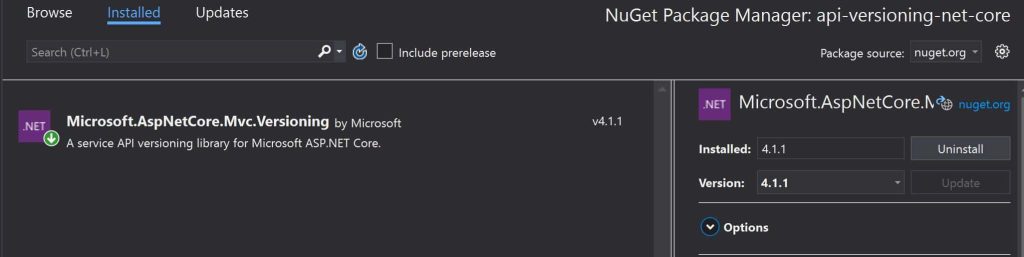
Add the NSwag Nuget package
PM> Install-Package NSwag.AspNetCore -Version <version>
OR
Install it through Nuget Package Manager,

Please use the latest available version.
Step2
NSwag – Enable API Versioning in API
Please enable API versioning using the below code in the ConfigureService method in Startup.cs,
The ApiVersioningOptions allows you to configure, customize, and extend the behaviors.
services.AddApiVersioning(config =>
{
config.ApiVersionReader = new UrlSegmentApiVersionReader();
config.ReportApiVersions = true;
});
Add Versioning ApiExplorer Nuget package
Please add below the ApiExplorer Nuget package
PM> Install-Package Microsoft.AspNetCore.Mvc.Versioning.ApiExplorer -Version 4.2.0
This NuGet package will be used for discovering metadata such as the list of API-versioned controllers and actions, their URLs, and allowed HTTP methods.
Let’s add an API explorer that is API version aware. We can specify the version format.
services.AddVersionedApiExplorer(option =>
{
option.GroupNameFormat = "VVV";
option.SubstituteApiVersionInUrl = true;
});
I have below a sample Controller with specific endpoints.
SnowForecastController
I have two methods decorated with version 1.0

RainForecastController
I have 1 method decorated with version 2.0

Automating API version detection and adding it to the NSwag list
Please define SwaggerDocument using API Version Descriptor by discovering metadata such as the list of API-versioned controllers and actions.

Let’s execute the swagger URL. You shall see all available versions will be listed below.

NSwag API Versioning output for v1 version
NSwag API Versioning output for the v1 version will be displayed below,

NSwag Swagger API Versioning output for v2 version
NSwag API Versioning output for v2 version will be displayed as below,

Do you have any comments or ideas or any better suggestions to share?
Please sound off your comments below.
Happy Coding !!
References :
Summary
We learned how to use NSwag to add swagger documentation with API Versioning support in this post. OpenAPI explains how to handle versioning as well as the standards and guidelines for RESTFul API descriptions. Using the Nswag Nuget package and tooling in ASP.NET Core, enabling OpenAPI documentation with version support is simple.
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.
IApiVersionDescriptionProvider how to Apply this in .net core 6 Progame.cs file
Where exactly do you implement the foreach loop?
Hi Elena- I have added within the ConfigureServices() method where I got the instance of IApiVersionDescriptionProvider as a service object. Thank for the query.
This is still not clear. Can you provide the source code for this part?