PyMongo – Insert datetime into Mongodb as ISODate
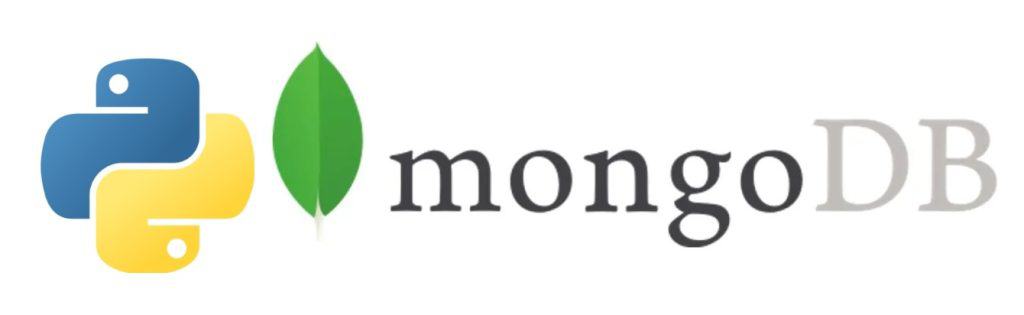
Today in this article, we will see how to perform PyMongo – Insert datetime as ISODate in Python.
We will use Mongo Driver called pymongo to insert the date in the collection in the ISO format.
Getting started
Here below is a sample schema or document we shall use for the date range query,
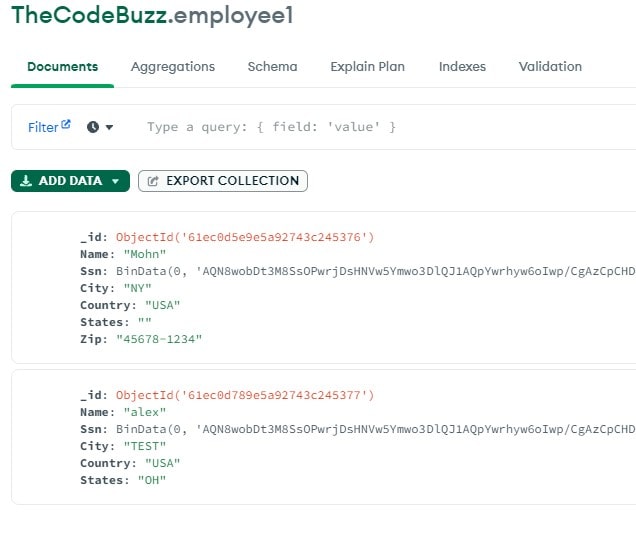
Install PyMongo package
Please install the PyMongo driver package to get support for the MongoClient connection class and properties.
For more details visit- https://api.mongodb.com/python/current
Add PyMongo Import statements
Please add below using namespaces,
from pymongo import MongoClient from datetime import datetime
If you need support for date time zone support in UTC, ETC, etc, please add below other import statements.
from datetime import datetime, tzinfo, timezone
Define MongoDB Connection
client = MongoClient('mongodb://localhost:27017/')
db = client['your_database']
collection = db['your_collection']
Python – Insert Date Time in ISO format
Define the date for the query
date = datetime(2023, 1, 1)
OR
Date time using time zone
date = datetime(2023, 1, 1, tzinfo=timezone.utc)
Note: When used with datetime, MongoDB by default uses ISO format to insert any date in the mongo collection.
Define the filter
filter = { //define any filter as needed }
Define the update operation
Please define the update operation (update_operation
) to fit your data structure and update requirements as needed.
Below we are setting the “Date” the date field with ISO date in all the documents in the collection.
# Define the update operation
update_operation = {
'$set': {
'ModifiedDate': date
}
}
Perform update_many to insert the ISO date
Let’s perform the update_many operations as below. update_many
in PyMongo permits you to update multiple documents that match a specified filter.
We simplification purposes, we will insert the ISO date in every document using update_many
.
result = collection.update_many({},update_operation)
Above I have defined an empty filter.

Do you have any comments or ideas or any better suggestions to share?
Please sound off your comments below.
Happy Coding !!
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.