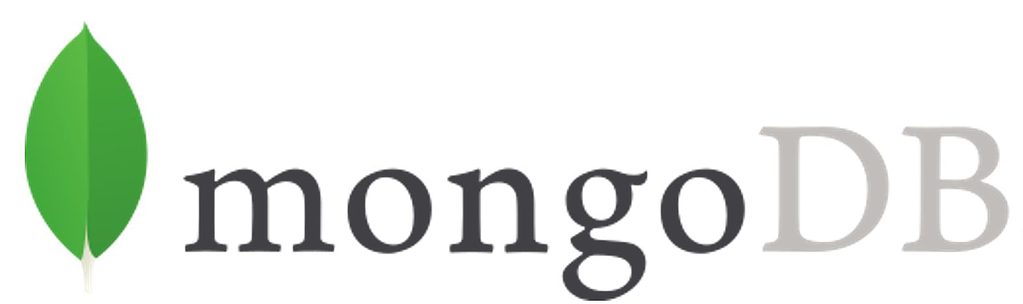
Today this article will see how to Update the MongoDB field using the value of another field.
This could be a legitimate requirement for you to add or update a Mongo field using the value of another field in MongoDB.
Today in this article, we will cover below aspects,
We shall see examples for MongoDB field query where we will update the existing field using values from another and in another scenario, we will create a new field using values of another field.
We already looked at a simple way of adding or updating a new field to the document in our previous MongoDB sample series.
We shall also see how to create a similar query using another language like C# mongo driver.
Getting started
Here below is a sample schema or document we shall use for field length query and will cover less than or greater than length query for field value “ZIP”.
Update MongoDB field using the value of another field
Here we will be using the below schema where the Zip field currently has 5 digits. We also have the Zip5 field and Zip4 field which are standard Zipcode-specified fields.
We will use Zip5 and Zip4 to derive the value and assign it to the Zip field
Source example
{
"_id": {
"$oid": "61ec0d5e9e5a92743c245376"
},
"Name": "JK",
"City": "NY",
"Country": "USA",
"States": "NY",
"Zip5": "67889",
"Zip4": "12345"
"Zip": "67889"
}
Below is how we will are expecting the Zip by performing Zip5+Zip4
{
"_id": {
"$oid": "61ec0d5e9e5a92743c245376"
},
"Name": "AK",
"City": "NY",
"Country": "USA",
"States": "NY",
"Zip5": "67889",
"Zip4": "12345"
"Zip": "67889-12345"
}
Pattern:
db.employee.updateMany({},
[{
$set: {
<target-field>: {
$toString: {
$concat: ["<expression1>", "<expression2>","<expression3>..."]
}
}
}
}]
)
Example:

Here is the output of the query,
Add a new MongoDB field using the value of another field
The above-used “Set” operator does perform even adding the new field if it doesn’t exist already.
Let’s say the above zip4 element is null or empty in such cases, you may want to use and append “00000” as zip4.
Below is how we can achieve this.
{
"Zip": "67889-0000"
}
Query Pattern
db.employee.updateMany({},
[{
$set: {
"Zip": {
$toString: {
$concat: [{
$toString: "$Zip"
}, {
$substrCP: ["-0000", 0, {
$subtract: [10, {
$strLenCP: {
$toString: "$Zip"
}
}]
}]
}]
}
}
}
}]
)
Kindly visit the below article for all examples using the C# MongoDB driver,
The above query can be extended for filters or conditional updates as required.
Do you have any comments or ideas or any better suggestions to share?
Please sound off your comments below.
Happy Coding !!
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.