C#.NET MySQL OPTIMIZE all tables – Guidelines

Today in this article, we will learn about C# .NET MySQL OPTIMIZE all tables. We will also see how to get all tables listed from MySQL and optimize each table programmatically using C#.NET.
As a developer or DBA (Database administrator) or tester, it’s important of validating the schema your database supports which includes all the tables and their structure.
Today, in this article, we will discuss below aspects,
Getting a list of tables in a database is a most useful query that most developers use.
Getting started
Create any .NET or .NET Core application. To keep it simple I shall be using the Console application however you can use any project template.
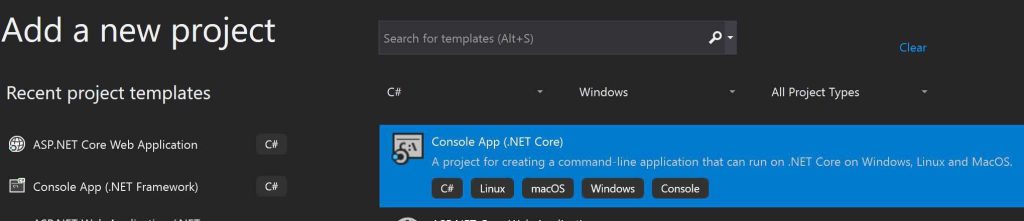
Add NuGet package – MySqlConnector
Please install the MySqlConnector Nuget package using Nuget Package Manager,
PM> NuGet\Install-Package Microsoft.Extensions.Configuration -Version 6.0.1
And
PM> NuGet\Install-Package MySqlConnector -Version 2.1.13
OR using .NET CLI
dotnet add package Microsoft.Extensions.Configuration --version 6.0.1
and
dotnet add package MySqlConnector --version 2.1.13
Command
Let us validate the with the most basic command as below. The following query will show all tables in a MySQL database:
SHOW TABLES;
Once you know the table to be optimized, use the below query to optimize the same
OPTIMIZE TABLE {table}
If you have multiple tables to be optimized, you can specify the table list as below,
OPTIMIZE TABLE { table1, table2, table3 }
Below is a sample C# query supporting the show tables,
MySqlCommand cmd = new MySqlCommand($"OPTIMIZE TABLE {table}", connection);
Optimizing the table using C# .NET Code
Optimizing the given table using C# .NET Code can be achieved as below,
Sample code,
try
{
connection.ConnectionString = con2;
connection.Open();
MySqlCommand cmd = new MySqlCommand($"OPTIMIZE TABLE {table}", connection);
cmd.ExecuteNonQuery();
}
}
catch (Exception ex)
{
}
connection.Close();
Where table is the table name to be optimized.
Optimizing All Tables using C# .NET Code
If you’re interested to optimize all the tables in the given database using C# .NET Code then please see the below code to achieve the same.
try
{
connection.ConnectionString = con2;
connection.Open();
string cmdStr = "show tables";
TableNames = MySqlTableCollections(connection, cmdStr);
foreach (string table in TableNames)
{
MySqlCommand cmd = new MySqlCommand($"OPTIMIZE TABLE {table}", connection);
cmd.ExecuteNonQuery();
}
}
catch (Exception ex)
{
}
connection.Close();
MySqlTableCollections method logic is defined as below,

Do you have any comments or ideas or any better suggestions to share?
Please sound off your comments below.
Happy Coding !!
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.