How to create Custom Converters for JSON serialization in .NET with example
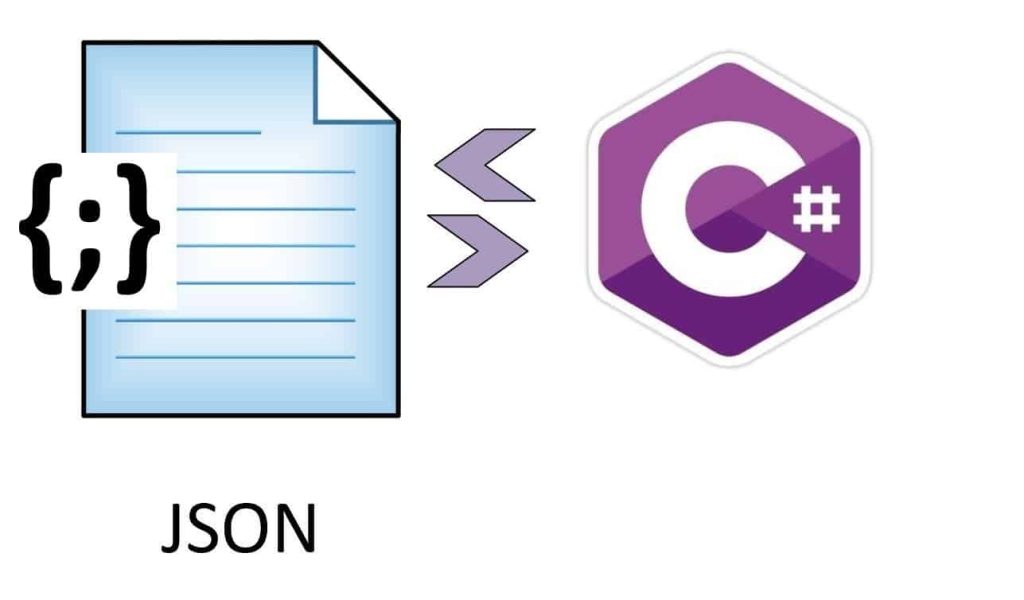
We shall Create custom Converters for JSON serialization in .NET with an example of a StringConverter.
We shall be following below high-level steps create a converter using a basic pattern,
- Create a Custom Converter (Derived from JsonConverter<T>)
- Override the Read method
- Override the Write method
- Register a Custom converter as a serializer option.
- Perform serialize/deserialize
There are mainly two patterns for creating any custom converter. We shall be covering the ‘Basic pattern‘ in this article. A ‘Factory pattern‘ useful for generic type conversion, we shall look into it in the next article.
As we know the new .NET /ASP.NET Core 3.1 framework has removed the dependency on JSON.NET and uses it’s own JSON serializer i.e ‘System.Text.Json‘.
As per specification and design, there are known constraints in the System.Text.Json serializer.
To avoid any challenges around data conversion best alternative is to write a custom converter.
Today we shall perform generic steps required to create basic custom Converters for JSON serialization and will also create StringConverter as an example.
Getting Started
Please create Any .NET application.
Create a Custom Converter
Create a Custom Converter class derived from JsonConverter<T>. Name it as appropriate.
Here T is the type to be serialized and deserialized which could be either any primitive type or complex type as needed.
Example:
Here I am creating a StringConverter to provide ability to serialize any Number as String.
public class StringConverter : JsonConverter<string>
{
.
.
}
Override the Read/Write method
Override the Read method and use Utf8JsonReader to read the token type as Number. If you find any numeric details deserialize it to the string type as below.
public override string Read(ref Utf8JsonReader reader, Type typeToConvert, JsonSerializerOptions options)
{
if (reader.TokenType == JsonTokenType.Number)
{
var stringValue = reader.GetInt32();
return stringValue.ToString();
}
else if (reader.TokenType == JsonTokenType.String)
{
return reader.GetString();
}
throw new System.Text.Json.JsonException();
}
Similarly Override the Write method as below,
public override void Write(Utf8JsonWriter writer, string value, JsonSerializerOptions options)
{
writer.WriteStringValue(value);
}
WriteStringValue method writes a string text value (as a JSON string) as an element of a JSON array.
Register a Custom converter as a serializer
Use JsonSerializerOptions to register the custom converter.
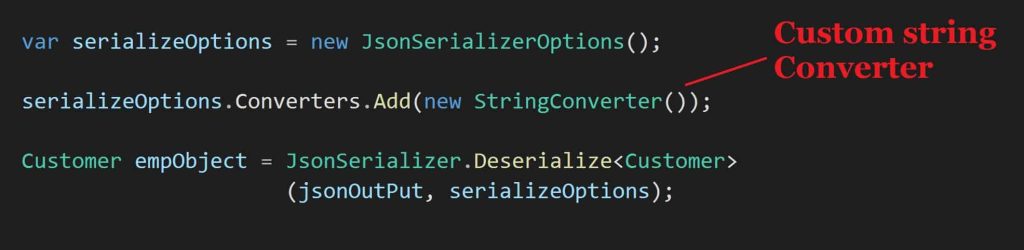
Perform serialize/deserialize
You are all set to use the above converter for performing serialization and deserialization. We shall be able to use JsonSerializer.Deserialize()..overloaded to pass additional string converter option.
Above converter shall be able to deserialize any Number to string as required for sterilization of target type i.e Customer
Sample JSON:
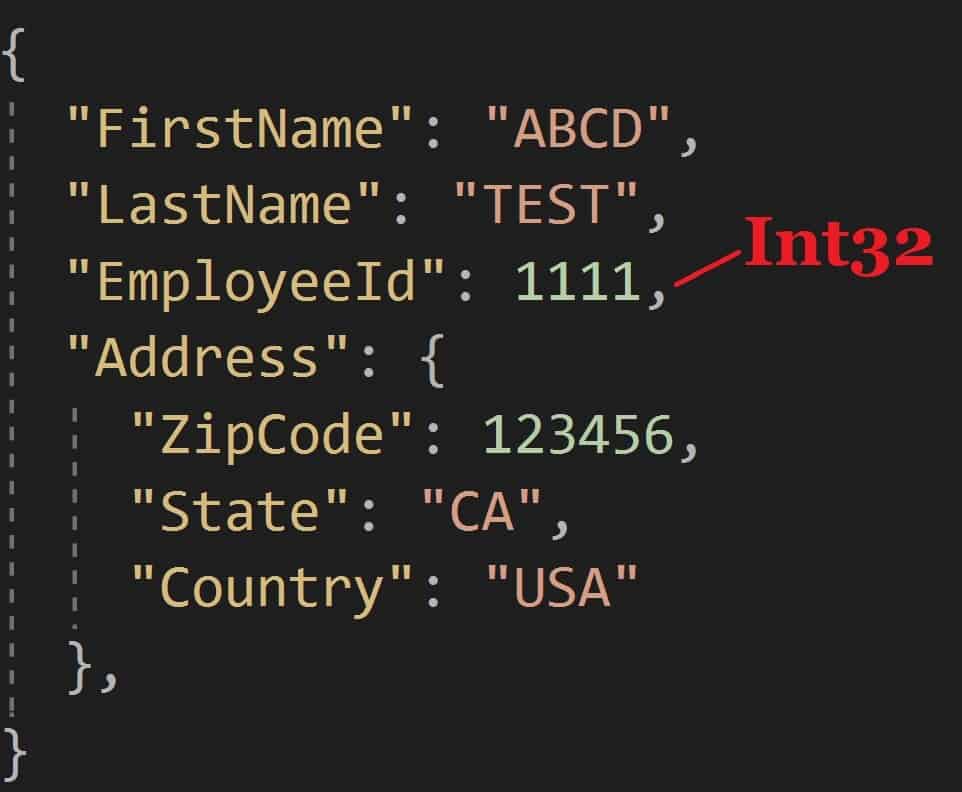
Target Customer Type is defined as below,
public class Customer
{
public string FirstName { get; set; }
public string LastName { get; set; }
public string EmployeeId { get; set; }
public Address Address { get; set; }
}
public class Address
{
public int ZipCode { get; set; }
public string State { get; set; }
public string Country { get; set; }
}
Finally JSON conversion is successful,

References:
Summary
Today in this article we learned how to create Custom Converters for JSON serialization in .NET (with StringConverter as an example). As per specification and design, there are known constraints in the System.Text.Json serializer. To address any custom serialization/deserialization requirement, we need to create a custom converter as per the need.
Do you have any comments or ideas or any better suggestions to share?
Please sound off your comments below.
Happy Coding !!
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.